Dynamic Keyframes
Dynamic Keyframes plugin for unreal engine streamlines procedural animation by modularizing animations into runtime-adjustable keyframes (AnimTasks) that are grouped and sequenced effortlessly. The system’s flexibility allows you to extend it with custom tasks and templates. This modular approach lets you drive any UObject—be it an Actor, Component, or custom object—without rewriting procedural logic.
Table of Contents
Where To buy
You can get the Dynamic Keyframes Plugin for Unreal Engine 5 on Fab.com
Watch the video
How To Use The Procedural Animation Templates
Once you have plugin installed, you’ll find the animation templates in the location Dynamic Keyframes Content/Blueprints/AnimTemplates.
These Blueprints are SceneComponents that you can add to any actor.
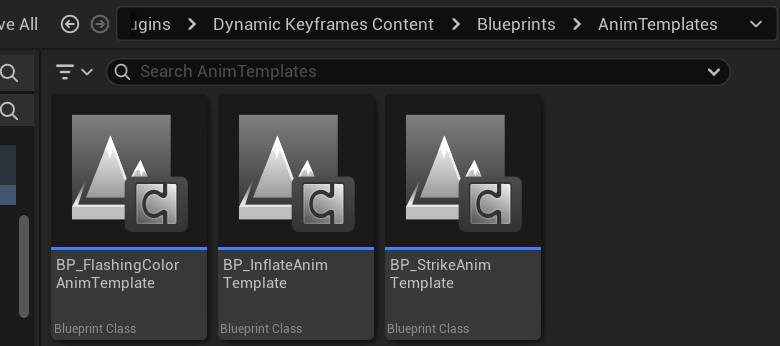
Add The Anim Template As A Component of Any Actor.
Create a new AActor Blueprint or open the example actors. The Example Actors are in the directory Dynamic Keyframes Content/Blueprints/ExampleActors.
Click the Add Component button on the Actor Blueprint and choose one of the AnimTemplates. For this example or explanation we’ll choose the BP_StrikeAnimTemplate componet.
This procedural anim template allows you to easily animate something that should strike, like lightning or a drum stick hitting a snare drum.
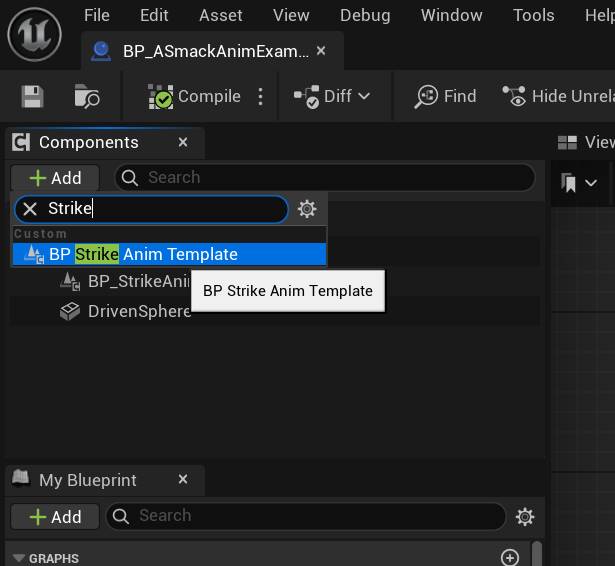
Subscribe to the AnimTemplates' Events
Every AnimTemplate should or will contain Events that the Owning Actor should subscribe to.
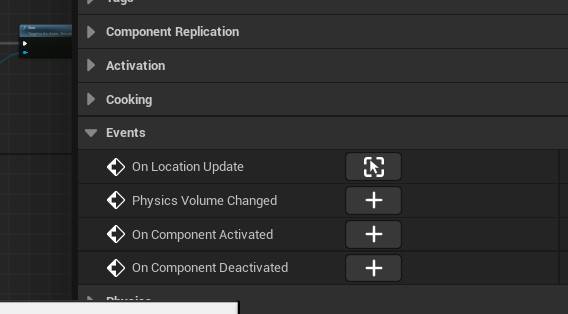
The StrikeAnimTemplate contains the the “OnLocationUpdate” event/delegate.
This event is fired on tick as the “Striking” animation occurs and allows you to update the Component, Actor or element that should do the “Striking”. In our Example Actor, we call the “SetWorldLocation” function on the Driven Sphere.
So as the procedural animation changes location, so will our visible sphere, which will give it the striking effect.
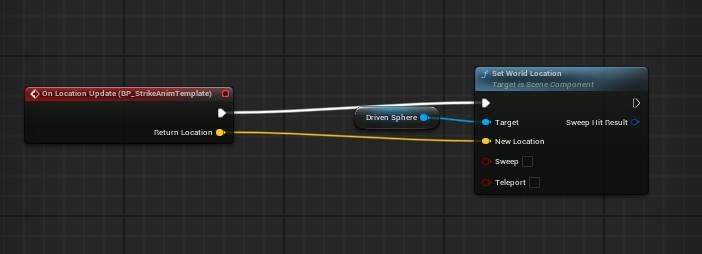
Setting the Template Properties.
A Procedural Anim Template can have its properties set on the details panel or procedurally at runtime. For example, you can change or set the StrikeLocation.

In the above we are changing or setting the StrikeAnimTemplate’s Strick Location and Return Location on Begin Play.
The Return Location is where to return to after striking.
You can also set the StrikeDuration. This is the amount of time it will take for to reach the target strike location and the “ReturnDuration” is the amount of time it will take to go back after striking.
All properties you see can be changed at runtime to create fully procedural animations or target strike locations.
Start the Procedural Anim Template
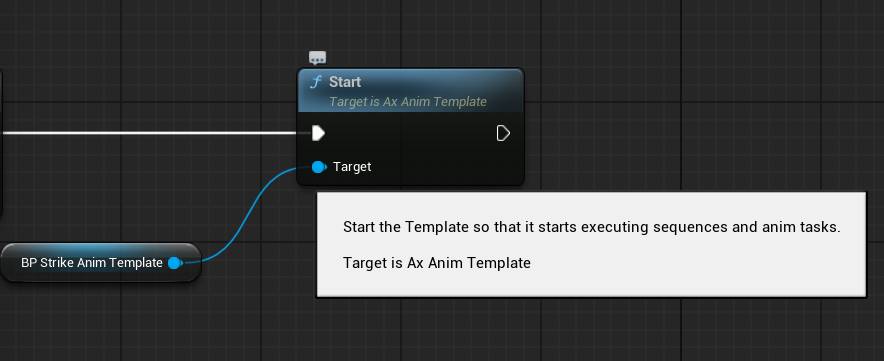
Once you’re done setting the properties, you must call the “Start” function on the AnimTemplate. This puts in a state where its ready to process anim tasks(explained below).
Triggering the Strike or Procedural Action.
AnimTemplates will usually have procedural action you can trigger at will. For example or Strike anim template, has the “Strike” trigger. This will make the animation strike the target location. You’ll want to call this function for example when a MIDI Note turns on so that your Drum Stick “Strikes” the snare drum or whatever you want to use the animation for.
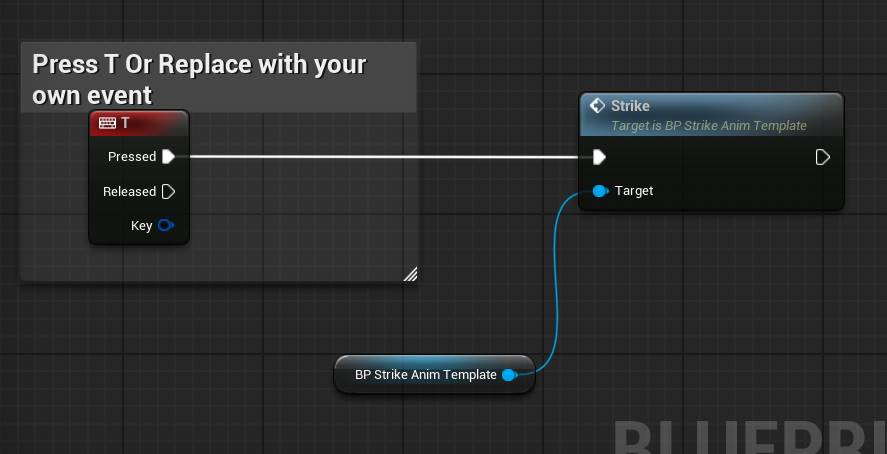
Other Templates: Inflate and Flashing Color
There’s other templates you can use. For example the InflateTemplate is used when you want to animate scale that inflates and then deflates when triggered.
The Flashing Color Template is to be used when you want to animate a color , like flashing lights when music plays.
The’re events and properties are similar to the Strike Anim Template component.
Creating your own Procedural Animation Templates
In order to create your own dynamic animation templates, you need to understand a few concepts which are explained below. It’s recommended you copy/duplicate the existing templates and customize them before attempting your own. Also the BP_StrikeAnimTemplate contains the documentaion and explaination of how a procedural animation template is made. Open it and read the comments.
Anim Task : The Dynamic Keyframes
The AnimTask class is the base class for dynamic or procedural animations in unreal engine. Each AnimTask is driven by a timeline and updates its properties—like duration, start/stop values, and evaluation curves—during runtime. Payload objects associated with an AnimTask receive the evaluated data, allowing for dynamic updates to any UObject.
Key Features
- Timeline Driven: Executes animations over a set duration.
- Dynamic Properties: Supports runtime modifications such as duration, start/stop values, and evaluation curves.
- Payload Support: Applies evaluated values to any associated UObject.
- Expandable: Create custom tasks (e.g., Spline-based, Material animations) by subclassing and overriding
Evaluate()
.
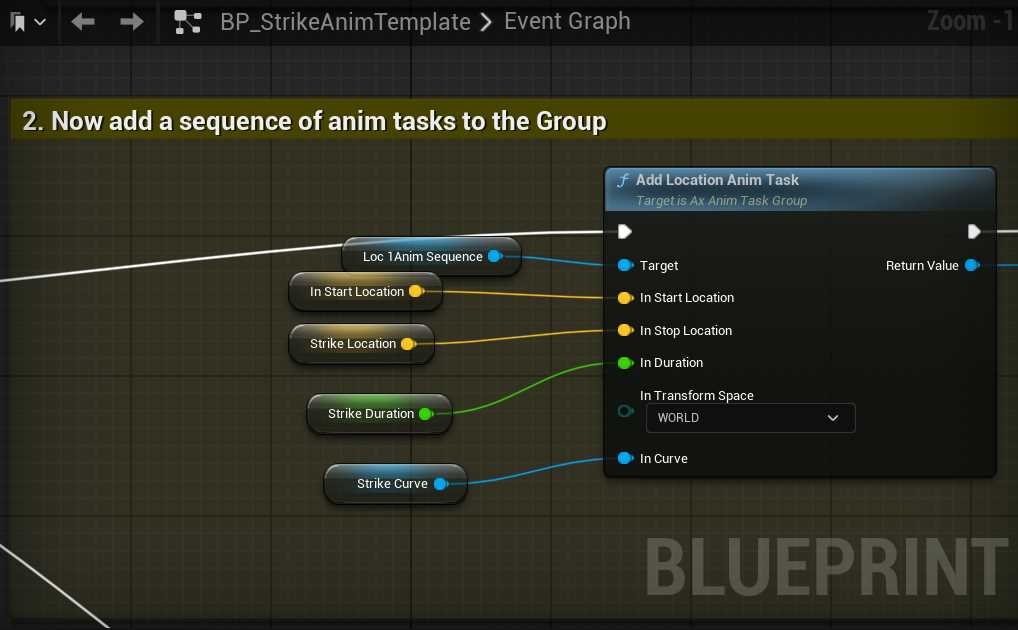
AnimTaskSequence – Sequencing Procedural Keyframes
The AnimTask Sequence queues AnimTasks and plays them in order. It supports auto-starting the next task when one completes, or manual progression. This does not mean we are hardcoding the animation like traditional keyframe based systems do. Remember the keyframes or their properties can still be changed from blueprints while the sequence is running.
Key Features
- Queue Execution: Tasks play sequentially.
- Auto/Manual Control: Option to automatically trigger subsequent tasks.
- Blueprint Integration: Exposed functions for adding, removing, and managing tasks.
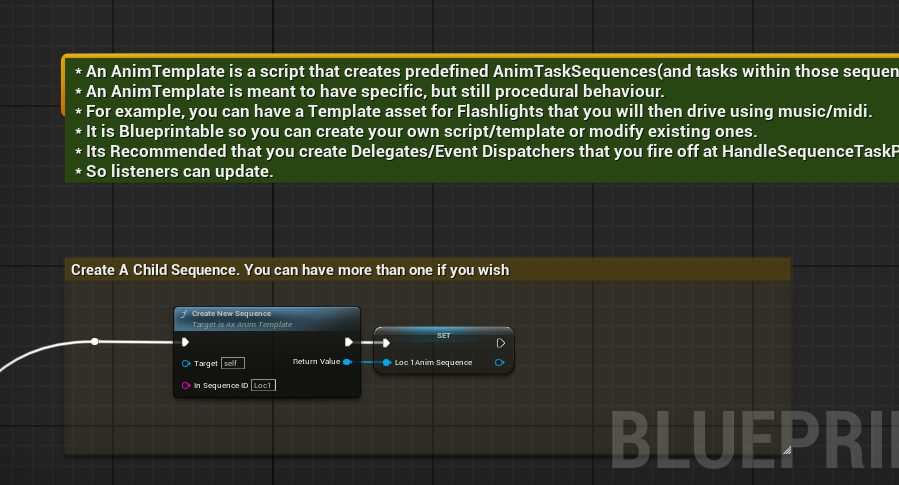
AnimTemplate – Reusable Animation Scripts
AnimTemplate is a Blueprintable component designed for authoring and reusing animation scripts. Templates manage multiple AnimTask sequences, each identified by unique IDs. You can create your own templates or customize the defaults, allowing your animation logic to be reused across various projects and scenarios.
Key Features
- Predefined Sequences: Organize and manage multiple AnimTask sequences by unique IDs.
- High Flexibility: Create or extend templates without constraints—drive any UObject with your procedural logic.
- Blueprint Events: Override native events like
InitTemplate
,HandleSequenceTaskPostEvaluate
, andHandleSequenceTaskComplete
for custom behavior. - Reusable Logic: Once an AnimTemplate or AnimTask is created, it can be applied to any actor, component, or UObject without additional scripting.
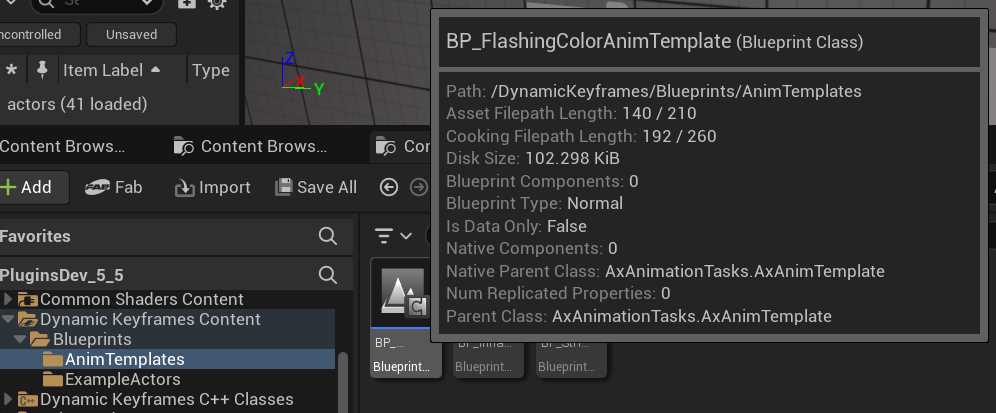
Creating Procedural Animation Assets : High level overview
The core workflow starts with an AnimTemplate asset. This asset is designed to be added to any Actor, driving animations across various components or even other actors. Here’s how the system ties together:
- AnimTasks: The basic building blocks. Each task represents a dynamic keyframe, with its own duration, start/stop values, and evaluation curve.
- AnimTaskSequence: A collection of AnimTasks that play sequentially. This sequence governs the order and flow of animations.
- AnimTemplate: The scriptable asset that bundles one or more AnimTaskSequences. It exposes parameters for configuration and provides delegates/Event Dispatchers (e.g., OnLocationUpdate) to allow you to hook into key animation events.
This setup enables you to author reusable, procedural animation assets. Once configured, an AnimTemplate can dynamically drive any UObject, making it easy to apply complex animations without rewriting procedural logic for every new Actor or component.